아카이브
페이드인, 페이드 아웃 연습 본문
페이드 아웃 만들기
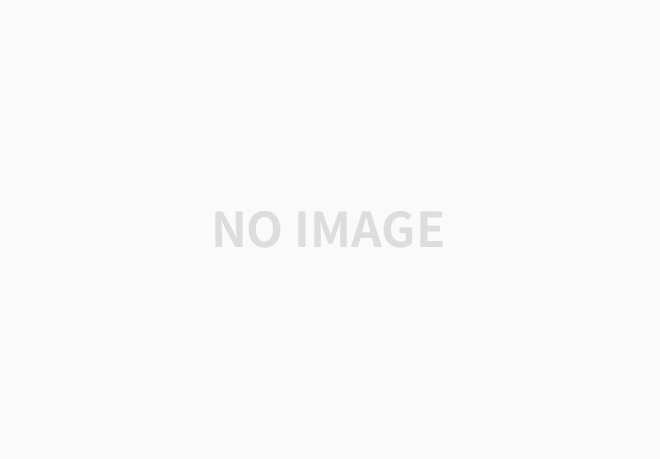
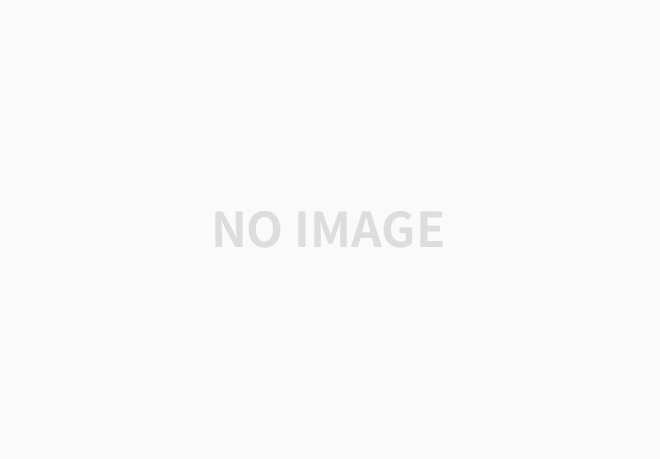
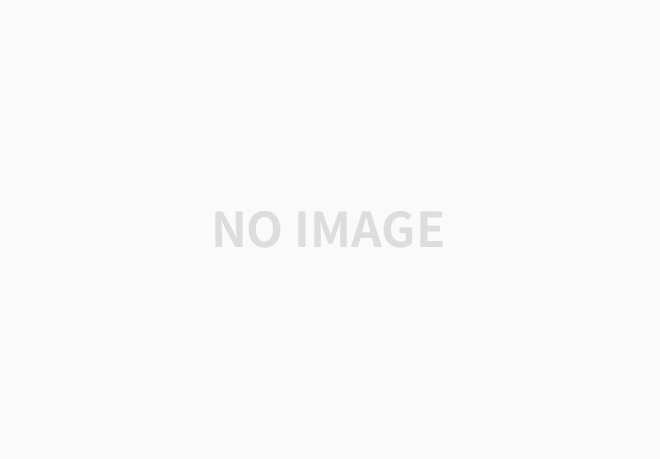
스크립트
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class Test_FadeOutMain : MonoBehaviour
{
[SerializeField]
private Image dim;
// Start is called before the first frame update
void Start()
{
this.StartCoroutine(this.FadeOut());
}
private IEnumerator FadeOut()
{
Color color = this.dim.color;
//점점 어두워지게
while (true)
{
color.a += 0.01f;
this.dim.color = color;
Debug.Log(this.dim.color.a);
if (this.dim.color.a >= 1)
{
break;
}
yield return null; //다음 프레임 시작
}
Debug.LogFormat("fadeout complete!");
}
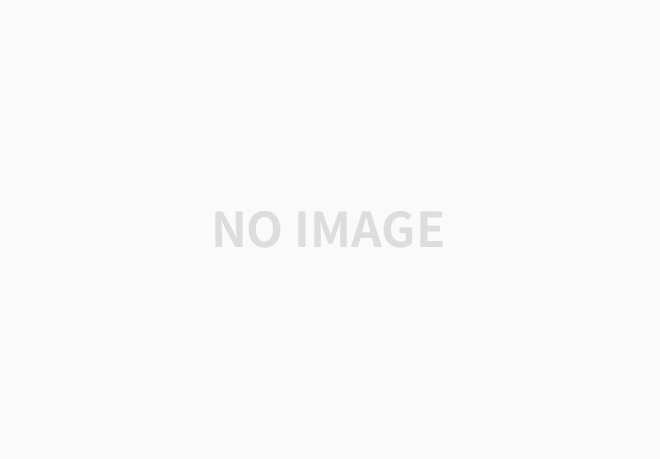
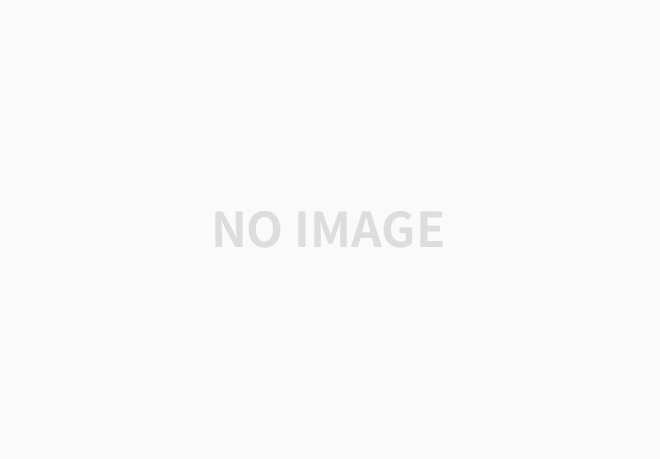
페이드인 만들기
페이드 아웃 만들 때와 비슷하지만 dim 의 알파값을 0이 아니라 최대로 맞춰야함
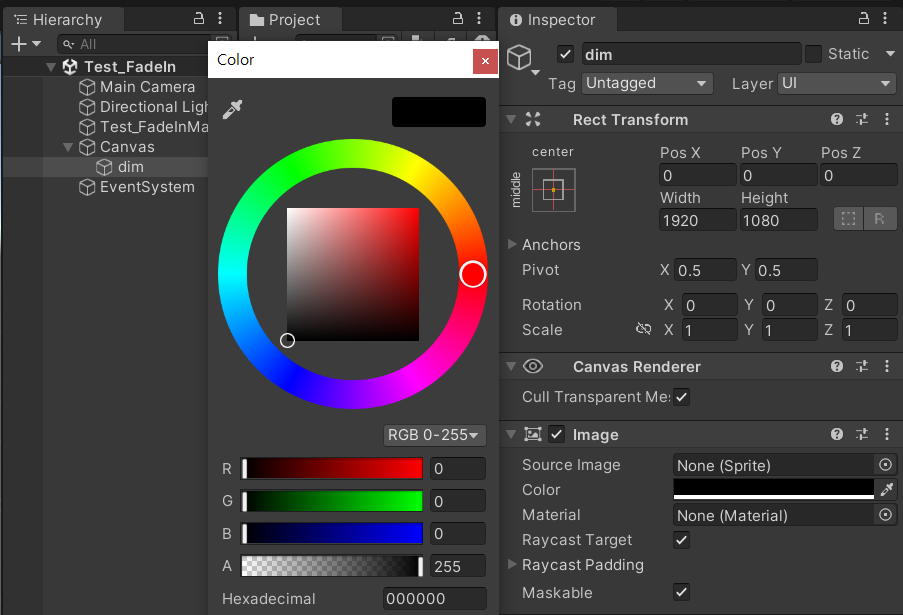
스크립트
FadeOut
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class Test_FadeOutMain : MonoBehaviour
{
[SerializeField]
private Image dim;
private System.Action onFadeOutComplete;
// Start is called before the first frame update
void Start()
{
//이벤트 추가
this.onFadeOutComplete = () => {
//씬전환
SceneManager.LoadScene("Test_FadeIn");
};
this.StartCoroutine(this.FadeOut());
}
private IEnumerator FadeOut()
{
Color color = this.dim.color;
//점점 어두워지게
while (true)
{
color.a += 0.01f;
this.dim.color = color;
Debug.Log(this.dim.color.a);
if (this.dim.color.a >= 1)
{
break;
}
yield return null; //다음 프레임 시작
}
Debug.LogFormat("fadeout complete!");
this.onFadeOutComplete(); //대리자 호출
}
FadeIn
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
using UnityEngine.UI;
public class Test_FadeInMain : MonoBehaviour
{
[SerializeField]
private Image dim;
private System.Action onFadeInComplete;
void Start()
{
//이벤트 추가
this.onFadeInComplete = () => {
Debug.Log("FadeIn complete!");
};
this.StartCoroutine(this.FadeIn());
}
private IEnumerator FadeIn()
{
Color color = this.dim.color;
while (true)
{
color.a -= 0.01f;
this.dim.color = color;
Debug.Log(this.dim.color.a);
if (this.dim.color.a <= 0)
{
break;
}
yield return null;
}
Debug.LogFormat("fadeout complete!");
this.onFadeInComplete(); //대리자 호출
}
}
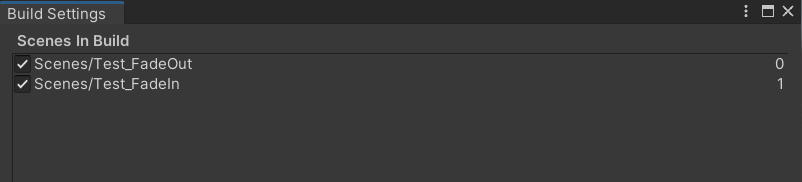
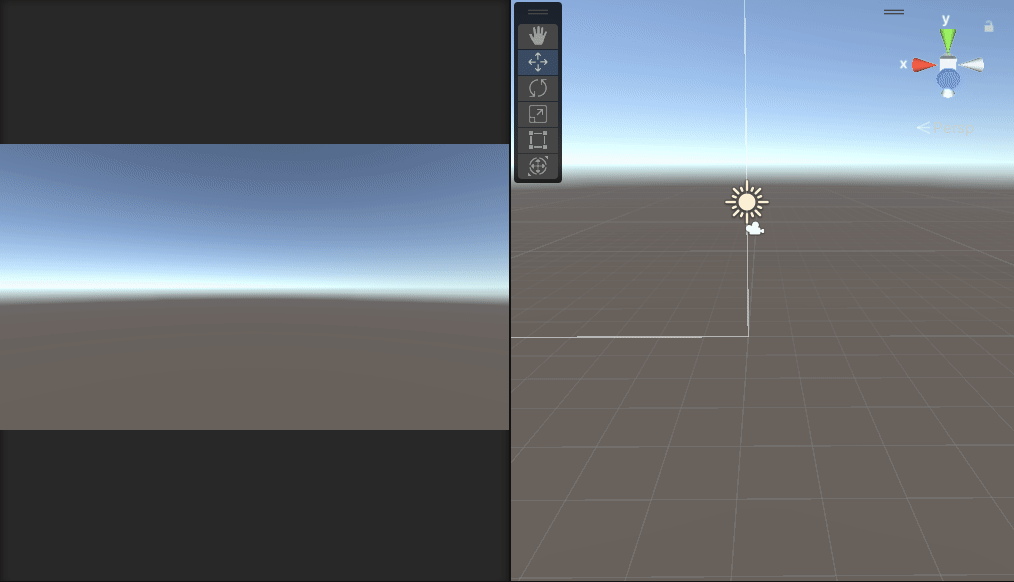
'유니티 기초' 카테고리의 다른 글
보스 만들기/시야 안에 타겟이 있는지 검사 (0) | 2023.08.14 |
---|---|
아이템 착용하기 (0) | 2023.08.11 |
클릭한 위치에 몬스터 생성/삭제 (0) | 2023.08.10 |
주말과제(Dog Knight) (1) | 2023.08.06 |
유니티짱 직선으로 움직이기 (0) | 2023.08.04 |